Class
Hierarchy
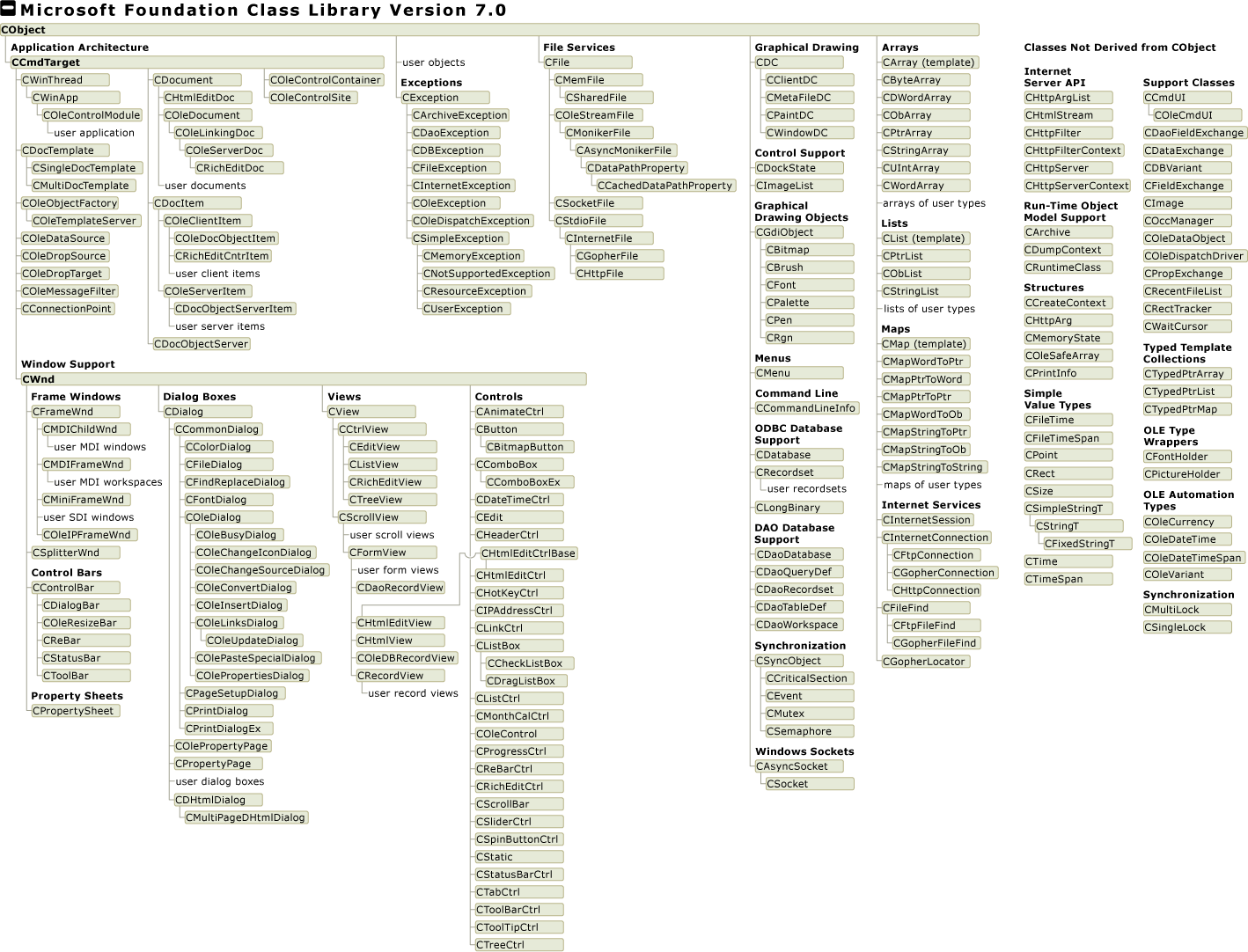
int DrawGLScene(GLvoid)
{
glClear(GL_COLOR_BUFFER_BIT |
GL_DEPTH_BUFFER_BIT);
glLoadIdentity();
glTranslatef(-1.5f,0.0f,-6.0f);
glColor3f(0.0f,0.0f,1.0f);
glBegin(GL_TRIANGLES);
glVertex3f( 0.0f, 1.0f,
0.0f);
glVertex3f(-1.0f,-1.0f, 0.0f);
glVertex3f( 1.0f,-1.0f,
0.0f);
glEnd();
glTranslatef(3.0f,0.0f,0.0f);
glBegin(GL_QUADS);
glColor3f(1.0f,0.0f,0.0f);
//red
glVertex3f(-1.0f, 1.0f, 0.0f);
glColor3f(0.0f,1.0f,0.0f);
//green
glVertex3f( 1.0f, 1.0f,
0.0f);
glColor3f(0.0f,0.0f,1.0f);
//blue
glVertex3f( 1.0f,-1.0f,
0.0f);
glColor3f(1.0f,0.0f,1.0f);
//pink
glVertex3f(-1.0f,-1.0f, 0.0f);
glEnd();
return TRUE;
}
CDC
Ever drawable object has an object of type CDC.
It comes with tens of utility functions for drawing on the screen.
TEXT Output
CPen* cp1 = new CPen(PS_INSIDEFRAME,2,
RGB(255,0,0));
CPen* cp2 = new CPen(PS_INSIDEFRAME,2,
RGB(0,255,0));
CPen* cp3 = new CPen(PS_INSIDEFRAME,2,
RGB(0,0,255));
CFont* fnt = new CFont();
fnt->CreateFont(
12,
// nHeight
0,
// nWidth
0,
// nEscapement
0,
// nOrientation
FW_NORMAL,
// nWeight
FALSE,
// bItalic
FALSE,
// bUnderline
0,
// cStrikeOut
ANSI_CHARSET,
// nCharSet
OUT_DEFAULT_PRECIS,
// nOutPrecision
CLIP_DEFAULT_PRECIS,
// nClipPrecision
DEFAULT_QUALITY,
// nQuality
DEFAULT_PITCH | FF_SWISS, // nPitchAndFamily
"Arial");
// lpszFacename
pDC->SelectObject(fnt);
pDC->TextOutW(10,
10, _T("Salam"));
Brush, Polygon
CBrush * wbrush = new CBrush(RGB(0, 0,
0));
CBrush * bbrush = new CBrush(RGB(255, 255,
255));
int cst = 30;
for (int i=0; i < 8; i++)
for (int j=0; j <
8; j++){
pDC->FillRect(CRect(cst+cst*i, cst+cst*j, cst*i+2*cst, cst*j+2*cst), (i+j)%2
== 0?wbrush:bbrush);
}
Ellipse
BOOL Ellipse(
int x1,
int y1,
int x2,
int y2
);
BOOL Ellipse(
LPCRECT lpRect
);
Polygon
BOOL Polygon(
LPPOINT lpPoints,
int nCount
);
// find the client area
CRect rect;
GetClientRect(rect);
// draw with a thick blue pen
CPen penBlue(PS_SOLID, 5,
RGB(0, 0,
255));
CPen* pOldPen =
pDC->SelectObject(&penBlue);
// and a solid red brush
CBrush brushRed(RGB(255,
0, 0));
CBrush* pOldBrush = pDC->SelectObject(&brushRed);
// Find the midpoints of the top, right,
left, and bottom
// of the client area. They will be the
vertices of our polygon.
CPoint pts[4];
pts[0].x =
rect.left + rect.Width()/2;
pts[0].y =
rect.top;
pts[1].x =
rect.right;
pts[1].y =
rect.top + rect.Height()/2;
pts[2].x =
pts[0].x;
pts[2].y =
rect.bottom;
pts[3].x =
rect.left;
pts[3].y =
pts[1].y;
// Calling Polygon() on that array will
draw three lines
// between the points, as well as an
additional line to
// close the shape--from the last point
to the first point
// we specified.
pDC->Polygon(pts, 4);
// Put back the old objects.
pDC->SelectObject(pOldPen);
pDC->SelectObject(pOldBrush);
What's that?
It's like an interrupt.
UINT SetTimer(UINT nIDEvent,
UINT nElapse,
void (CALLBACK EXPORT*
lpfnTimer)(HWND, UINT, UINT, DWORD));
example
BOOL CRandShapesDlg::OnInitDialog()
{
CDialog::OnInitDialog();
// Set the icon for this
dialog. The framework does this automatically
// when the application's
main window is not a dialog
SetIcon(m_hIcon, TRUE); // Set big icon
SetIcon(m_hIcon, FALSE); // Set small icon
// TODO: Add extra
initialization here
DlgWidth =
GetSystemMetrics(SM_CXSCREEN);
DlgHeight =
GetSystemMetrics(SM_CYSCREEN);
SetWindowPos(&wndTopMost, 0, 0, DlgWidth, DlgHeight,
SWP_SHOWWINDOW);
SetTimer(1, 200, 0);
return TRUE; //
return TRUE unless you set the focus to a control
}
its sends a WM_TIMER message to the application.
kill it
CWnd::KillTimer()